Next.js is a powerful and popular framework for building React applications. It is a minimalistic yet feature-rich framework that allows developers to build server-side rendered (SSR) and static websites using React components. Next.js offers a range of benefits, making it a go-to choice for many web developers.
Next.js is a versatile framework that combines the power of React with server-side rendering and static site generation. Its features enhance performance, improve developer productivity, and provide excellent SEO capabilities. Whether you’re building a simple website or a complex web application, Next.js is a valuable tool to consider.
One of the key advantages of Next.js is its built-in support for server-side rendering. SSR enables faster page loads by rendering the initial HTML on the server before sending it to the client. This improves performance and ensures that search engines can crawl and index content effectively, resulting in better SEO.
Additionally, Next.js provides an excellent developer experience by offering features such as automatic code splitting, hot module replacement, and server-side rendering out of the box. These features make development more efficient and allow developers to focus on building high-quality user interfaces.
Another noteworthy feature of Next.js is its support for static site generation (SSG). This allows developers to pre-render pages at build time, generating static HTML files that can be served to the client. Static site generation not only enhances performance but also simplifies deployment by eliminating the need for a server at runtime.
Next.js also supports client-side rendering (CSR) for dynamic content. With its dynamic routing capabilities, Next.js enables smooth navigation between pages without requiring a full-page refresh.
From an SEO perspective, Next.js excels due to its server-side rendering and static site generation capabilities. Search engines can easily crawl and index the pre-rendered HTML, leading to improved visibility and higher rankings in search results. Moreover, Next.js supports essential SEO features like customizable meta tags, canonical URLs, and efficient handling of redirects.
Why you should consider incorporating Next.js into your React projects
There are several compelling reasons why you should consider incorporating Next.js into your React projects. Let’s explore some of the key advantages:
- Server-Side Rendering (SSR): Next.js provides built-in support for server-side rendering, allowing you to render React components on the server before sending them to the client. SSR improves initial page load times, enhances performance, and ensures search engines can effectively crawl and index your content, resulting in better SEO.
- Static Site Generation (SSG): Next.js also offers static site generation capabilities, allowing you to pre-render pages at build time. This generates static HTML files that can be served to the client, providing improved performance and simplifying deployment by eliminating the need for a server at runtime.
- Developer Experience: Next.js enhances the developer experience by providing features like automatic code splitting, hot module replacement, and server-side rendering out of the box. These features streamline development, boost productivity, and enable developers to focus on creating high-quality user interfaces.
- React Ecosystem Integration: Next.js is specifically designed for React projects, making it a natural fit within the React ecosystem. It leverages the power of React, allowing you to build robust web applications with ease.
- SEO-Friendly: With server-side rendering and static site generation, Next.js ensures that search engines can crawl and index your website effectively. It supports essential SEO features like customizable meta tags, canonical URLs, and efficient handling of redirects, enabling better visibility and higher rankings in search results.
- Community and Support: Next.js has a vibrant and active community of developers, providing a wealth of resources, tutorials, and support. This active community ensures that you can find help and guidance whenever you need it.
- Flexibility and Scalability: Next.js offers flexibility in terms of deployment options. Whether you choose to deploy your application as a server-rendered application or a statically generated site, Next.js can accommodate both scenarios. It also provides built-in support for API routes, enabling seamless integration with backend services.
By incorporating Next.js into your React projects, you can leverage its powerful features, improve performance, enhance SEO, and enjoy a robust developer experience. Whether you’re building a small website or a large-scale web application, Next.js is a versatile framework that can elevate your React projects to the next level.
Create a new Next.js Project
You clone the started project from the link given below:
Creating a new Next.js project is a straightforward process. Here’s a step-by-step guide to help you get started:
Install Node.js
Ensure that you have Node.js installed on your machine. You can download the latest version from the official Node.js website and follow the installation instructions for your operating system.
Create a New Next.js Project
Open your terminal or command prompt and navigate to the directory where you want to create your Next.js project.
Initialize a New Next.js Project
Run the following command to initialize a new Next.js project using the official Next.js CLI (Command Line Interface)
npx create-next-app next-starter-project
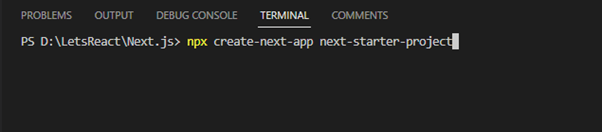
Replace “next-starter-project” with the desired name for your project. The npx command allows you to run the CLI without installing it globally. There are some options that you will be provided while setting up the new project. The options that I selected are as follows:

Once the project has been created Wait for the Initialization, The CLI will set up a new Next.js project for you, installing the necessary dependencies and configuring the project structure. This process may take a few moments, so be patient. Navigate to the Project Directory: Once the project initialization is complete, navigate to the newly created project directory by running the following command:
cd next-starter-project

Start the Development Server: To start the Next.js development server and run your project locally, use the following command:
npm run dev
This command will compile your Next.js project and start the development server at http://localhost:3000. You can access your project by opening this URL in your web browser.
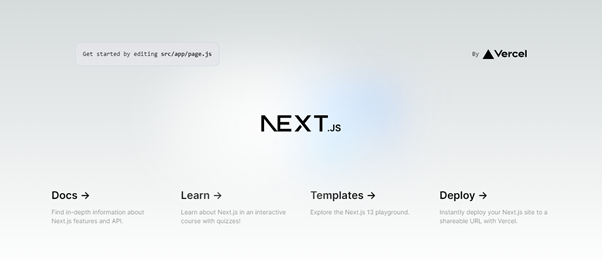
Congratulations! You have successfully created a new Next.js project. You can now start building your application by editing the files within the project directory. The Next.js framework provides a robust structure and offers features like server-side rendering and automatic hot module replacement to enhance your development experience.
Next.js Project Structure
The Next.js project structure follows a convention that provides a logical organization of files and directories. Understanding the project structure will help you navigate and work effectively with Next.js projects. Here is an overview of the main directories and files typically found in a Next.js project:

- .next/: This directory is automatically generated when you run the Next.js development server or build your project for production. It contains the compiled and optimized files for your Next.js application, including server-side rendered pages, static assets, and other build artifacts.
- node_modules/: This directory contains all the dependencies required by your Next.js project. It is created and populated when you install packages using a package manager like npm or yarn.
- pages/: This directory is a crucial part of Next.js. It contains your application’s pages, which represent the different routes of your website or application. Each file in this directory represents a page, and the file name determines the URL path. For example, pages/index.js corresponds to the root route /, and pages/about.js corresponds to /about.
- public/: This directory is used to store static assets that are directly accessible by the client. Files placed in the public/ directory can be referenced using relative URLs, starting from the root of the website. For example, an image placed in public/images/logo.png can be accessed via /images/logo.png.
- styles/: This directory is not present by default, but you can create it to organize your CSS stylesheets or any other styling files. You can import these stylesheets into your components or pages. Next.js supports various styling approaches, including CSS modules, CSS-in-JS libraries like styled components, and global CSS files.
- components/: This directory is not created by default but is commonly used to store reusable React components that are shared across multiple pages or sections of your application. It’s a good practice to organize your components in this directory to promote code reusability and maintainability.
- next.config.js: This file is used to configure Next.js and customize its behavior. You can modify various aspects of your Next.js project, such as webpack configuration, environment variables, asset optimization, and more, by adding configurations to this file.
- package.json: This file is a standard part of any Node.js project. It includes metadata about your project and lists all the project dependencies, scripts, and other project-specific configurations. You can also define custom scripts in the scripts section of this file to automate common tasks.
You clone the started project from the link given below:
Conclusion
We discussed Next.js, a powerful framework for building React applications. We highlighted its benefits, such as server-side rendering (SSR) and static site generation (SSG), which improve performance and SEO. We then provided a step-by-step guide on how to create a new Next.js project and explained the Next.js project structure, covering directories like pages, public, and components. Overall, Next.js offers a robust development experience and strong SEO capabilities, making it a valuable choice for React projects.
Some articles to learn more about React
Next.js Pages, Layouts, and Custom Header
Demystifying Next.js Routing: Dynamic Navigation and SEO Optimization
Deploy React Application on IIS Server
Why use React?
How to Improve React Performance with Lazy Loading?
2 Replies to “Next.js: The Powerhouse Framework for React Projects”